Array continues, pointer starts
1. Get the array length
Array length You can use the sizeof operator to get the length of the array, for example:
int numbers[] = {1, 2, 3, 4, 5}; int length = sizeof(numbers) / sizeof(numbers[0]); //sizeof calculates the byte length; the total number of numbers is 5*4=20 bytes;
pay attention:
Array memory is continuous
The array is a whole, and its memory is continuous; that is, the array elements are next to each other without any gaps between them. The following figure demonstrates the storage situation of int a[4];
in memory:
“Array memory is contiguous” is important, so I used a big title to emphasize it. Contiguous memory provides convenience for pointer operations (accessing array elements through pointers) and memory processing (copying, writing, etc. of the entire memory), which allows the array to be used as a cache (a piece of memory that temporarily stores data). You may not understand this sentence for the time being
2. Array name
In C language, the array name represents the address of the array, that is, the address of the first element of the array. When we declare and define an array, the array name represents the address of the array.
For example, in the following code:
int myArray[5] = {10, 20, 30, 40, 50};
Here, myArray is the array name, which represents an array of integer type, containing 5 elements. myArray also represents the address of the array, that is, the address of the first element.
The array name itself is a constant pointer, which means that its value cannot be changed. Once determined, it cannot point to other places.
We can use the & operator to get the address of the array as follows:
int myArray[5] = {10, 20, 30, 40, 50}; int *ptr = & amp;myArray[0]; // Or write directly int *ptr = myArray;
Three. Two-dimensional array
Introduction: Think about this question.
There are 5 people in a study group, and each person has test scores in 3 courses. Find the average score of each subject and the overall average score of the group.
– | Math | Chinese | English |
---|---|---|---|
Zhang Tao (No. 1) | 80 | 75 | 92 |
Wang Zhenghua (No. 2) | 61 | 65 | 71 |
Li Lili (No. 3) | 59 | 63 | 70 |
Zhao Quanquan (No. 4) | 85 | 87 | 90 |
Zhou Mengzhen (No. 5) | 76 | 77 | 85 |
First of all, the most primitive way is to count the scores one by one according to the table.
Of course it can be like this. If I want to query a classmate’s score for a subject one day in the future, I need to store this table. At this time, it will be very troublesome to use a one-dimensional array, so the two-dimensional array was born. .
1. Definition of two-dimensional array
The general form of a two-dimensional array definition is:
dataType arrayName[length1][length2];
Among them, dataType is the data type, arrayName is the array name, length1 is the length of the first dimension subscript, and length2 is the length of the second dimension subscript. For example:
int a[3][4];
A two-dimensional array with 3 rows and 4 columns is defined, with a total of 3×4=12 elements, and the array name is a, that is:
a[0][0], a[0][1], a[0][2], a[0][3] a[1][0], a[1][1], a[1][2], a[1][3] a[2][0], a[2][1], a[2][2], a[2][3]
If you want to represent the element in row 2 and column 1, you should write a[2][1].
I just told you that one-dimensional arrays are stored continuously in memory, and two-dimensional arrays are actually also stored continuously. Now think about how to store two-dimensional arrays in memory?
2. Initialize two-dimensional array
Multidimensional arrays can be initialized by specifying a value for each row within parentheses. Below is an array with 3 rows and 4 columns.
int a[3][4] = { {0, 1, 2, 3} , /* Initialize the row with index number 0 */ {4, 5, 6, 7} , /* Initialize the row with index number 1 */ {8, 9, 10, 11} /* Initialize the row with index number 2 */ };
Internally nested parentheses are optional, and the following initialization is equivalent to the above:
int a[3][4] = {0,1,2,3,4,5,6,7,8,9,10,11};
3. Access two-dimensional array
As we all know, one-dimensional arrays access the elements stored in the array through subscripts. Similar to one-dimensional arrays, two-dimensional arrays are also accessed through the following table.
What is the subscript of this array 5?
int a[3][4] = { {0, 1, 2, 3} , /* Initialize the row with index number 0 */ {4, 5, 6, 7} , /* Initialize the row with index number 1 */ {8, 9, 10, 11} /* Initialize the row with index number 2 */ };
Finally we consider the original question:
There are 5 people in a study group, and each person has test scores in 3 courses. Find the average score of each subject and the overall average score of the group.
– | Math | Chinese | English |
---|---|---|---|
Zhang Tao (No. 1) | 80 | 75 | 92 |
Wang Zhenghua (No. 2) | 61 | 65 | 71 |
Li Lili (No. 3) | 59 | 63 | 70 |
Zhao Quanquan (No. 4) | 85 | 87 | 90 |
Zhou Mengzhen (No. 5) | 76 | 77 | 85 |
First we can define a two-dimensional array to store:
int a[5][3]; //A two-dimensional array used to save the scores of each student in each subject
In the second step, how should we store the data in the table into an array (modeled after a one-dimensional array)
How should we calculate the average score after it is stored? Think about it (use subscripts to access)
#include <stdio.h> int main(){<!-- --> int i, j; //two-dimensional array subscript int sum = 0; //Total score of the current subject int average; //total average score int v[3]; //Average scores for each subject int a[5][3]; //A two-dimensional array used to save the scores of each student in each subject printf("Input score:\ "); for(i=0; i<3; i + + ){<!-- --> for(j=0; j<5; j + + ){<!-- --> scanf("%d", & amp;a[j][i]); //Enter each student's scores in each subject sum + = a[j][i]; //Calculate the total score of the current subject } v[i]=sum/5; // Average score of the current subject sum=0; } average = (v[0] + v[1] + v[2]) / 3; printf("Math: %d\ C Languag: %d\ English: %d\ ", v[0], v[1], v[2]); printf("Total: %d\ ", average); return 0; }
After talking about two-dimensional arrays, do you still remember that team leader Ren told everyone about the strcpy function in a question he wrote to familiarize everyone with the use of ida, which mentioned strings.
4. String
First of all, everyone knows that character data is represented by char. So what should I do if I want to input “Hello HuiHe” and output it on the screen? It cannot be said that I define a lot of char and then give them complex values H, e, l in turn. ,l,o, ,H,u,i,H,e, this is too complicated.
There is a data type string in all C languages, and the header file #include
1. String input
In C language, there are two functions that allow users to enter strings from the keyboard, they are:
- scanf(): Input a string through the format control character
%s
. In addition to strings, scanf() can also input other types of data. - gets(): Enter strings directly, and only strings can be entered.
However, there is a difference between scanf() and gets():
- When scanf() reads a string, it is separated by spaces. When it encounters a space, it considers the current string to end, so it cannot read a string containing spaces.
- gets() considers that spaces are also part of the string. It only considers that the string input ends when the Enter key is encountered. Therefore, no matter how many spaces are entered, as long as the Enter key is not pressed, it is a Complete string. In other words, gets() is used to read an entire line of string.
#include <stdio.h> int main(){<!-- --> char str1[30] = {<!-- -->0}; char str2[30] = {<!-- -->0}; char str3[30] = {<!-- -->0}; //gets() usage printf("Input a string: "); gets(str1); //scanf() usage printf("Input a string: "); scanf("%s", str2); scanf("%s", str3); printf("\ str1: %s\ ", str1); printf("str2: %s\ ", str2); printf("str3: %s\ ", str3);
str3: The first string input by JavaScript is completely read by gets() and stored in str1. The first half of the second input string is read by the first scanf() and stored in str2, and the second half is read by the second scanf() and stored in str3.
Note that scanf() needs the address of the data when reading data. This is constant, so for variables of int, char, float and other types, & amp;
to get their addresses. But in this code, we only gave the name of the string, but did not add & amp;
in front. Why is this? Because string names or array names are generally converted into addresses during use, adding &
is unnecessary and may even cause errors. //Pointers will be explained in detail later
2. String output
In C language, there are two functions that can output strings on the console (display), they are:
- puts(): Outputs a string and automatically wraps the line. This function can only output strings.
- printf(): Outputs a string through the format control character
%s
, and cannot automatically wrap lines. In addition to strings, printf() can also output other types of data.
#include <stdio.h> int main(){<!-- --> char str[] = "http://c.biancheng.net"; printf("%s\ ", str); //Output through string name printf("%s\ ", "http://c.biancheng.net"); //Direct output puts(str); //Output through string name puts("http://c.biancheng.net"); //Direct output return 0; }
There are a large number of functions in C for manipulating strings:
Serial number | Function & amp; Purpose | |
---|---|---|
1 | strcpy(s1, s2); Copy string s2 to String s1. | |
2 | strcat(s1, s2);< /strong> Concatenate string s2 to the end of string s1. | |
3 | strlen(s1); Returns the length of string s1. | |
4 | strcmp(s1, s2);< /strong> If s1 and s2 are the same, return 0; if s1 |
|
5 | strchr(s1, ch);< /strong> Returns a pointer pointing to the first occurrence of character ch in string s1. | |
6 | strstr(s1, s2);< /strong> Returns a pointer pointing to the first occurrence of string s2 in string s1. |
Everyone can go down and try it.
Pointer
Introduction:
All data in the computer must be placed in memory. Different types of data occupy different numbers of bytes. For example, int occupies 4 bytes and char occupies 1 byte. In order to access these data correctly, each byte must be numbered, just like a house number or an ID number. The number of each byte is unique, and a certain byte can be accurately found based on the number.
The following figure is the number of each byte in 4G memory (expressed in hexadecimal):
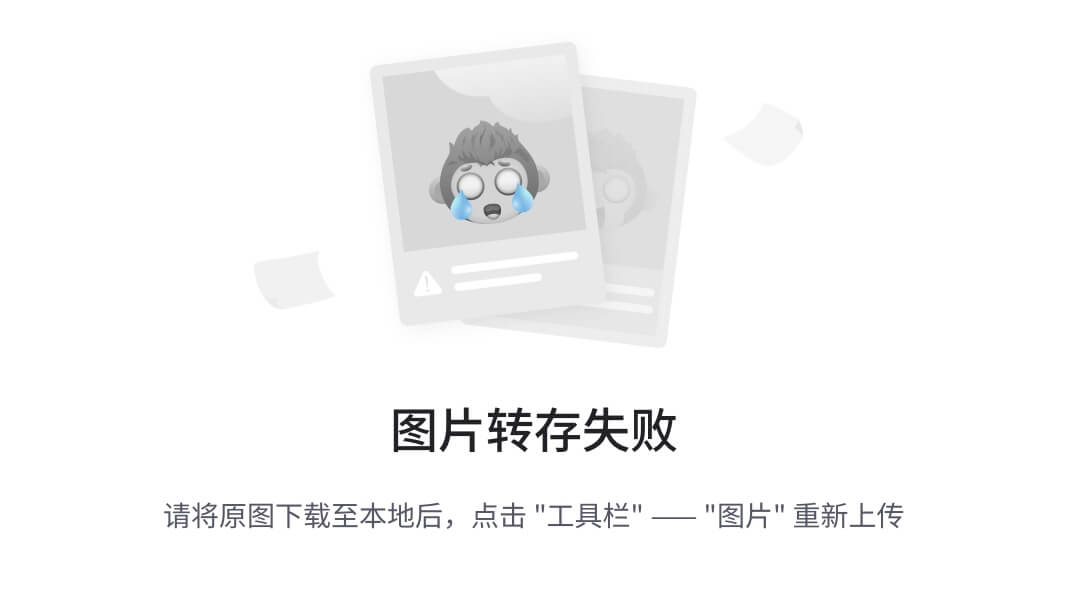{ int a = 100; char str[20] = "c.biancheng.net"; printf("%#X, %#X\ ", & amp;a, str); return 0; }
Everything is an address
C language uses variables to store data and functions to define a piece of code that can be reused. They must eventually be placed in memory before they can be used by the CPU.
Both data and code are stored in memory in binary form, and the computer cannot distinguish from the format whether a certain piece of memory stores data or code. When a program is loaded into memory, the operating system will assign different permissions to different memory blocks. The memory block with read and execute permissions is the code, and the memory block with read and write permissions (or maybe only read permissions) Memory blocks are data.
1. Definition and use of pointer variables
The address of data in memory is also called a pointer. If a variable stores a pointer to data, we call it a pointer variable. In C language, a variable is allowed to store a pointer, which is called a pointer variable. The value of a pointer variable is the address of a certain piece of data. Such data can be an array, a string, a function, or another ordinary variable or pointer variable.
Next tell the meaning of the pointer
Now suppose there is a variable c of type char, which stores the character K’ (the ASCII code is the decimal number 75) and occupies the memory at address 0X11A (the address is usually expressed in hexadecimal). There is also a pointer variable p, whose value is 0X11A, which is exactly equal to the address of variable c. In this case, we say that p points to c, or that p is a pointer to variable c.
Define pointer variables
Defining pointer variables is very similar to defining ordinary variables, but an asterisk *
must be added in front of the variable name. The format is:
datatype *name;
or
datatype *name = value;
*
indicates that this is a pointer variable, and datatype
indicates the type of data pointed to by the pointer variable.
int *p1; int a = 100; int *p_a = & amp;a;
When defining the pointer variable p_a, initialize it and assign the address of variable a to it. At this time, p_a points to a. It is worth noting that p_a requires an address, and the address character & amp;
must be added in front of a, otherwise it is incorrect.
Like ordinary variables, pointer variables can also be written multiple times. You can change the value of the pointer variable at any time as long as you want. Please see the following code:
//Define ordinary variables float a = 99.5, b = 10.6; char c = '@', d = '#'; //Define pointer variable float *p1 = & amp;a; char *p2 = &c; //Modify the value of the pointer variable p1 = &b; p2 = &d;
*
is a special symbol indicating that a variable is a pointer variable. *
must be included when defining p1 and p2. When assigning values to p1 and p2, since we already know that it is a pointer variable, there is no need to add *
. You can use pointer variables like ordinary variables later. In other words, *
must be used when defining a pointer variable, and *
cannot be used when assigning a value to a pointer variable.
It should be emphasized that the types of p1 and p2 are float*
and char*
respectively, not float
and char
, they are completely different data types, readers should pay attention.
Getting data through pointer variables
The pointer variable stores the address of the data. The data at the address can be obtained through the pointer variable. The format is:
*pointer;
The *
here is called the pointer operator, which is used to obtain data at a certain address. Please see the following example:
#include <stdio.h>int main(){<!-- --> int a = 15; int *p = &a; printf("%d, %d\ ", a, *p); //Both methods can output the value of a return 0;} ```c ```C In addition to obtaining data in memory, pointers can also modify data in memory, for example: #include <stdio.h> int main(){<!-- --> int a = 15, b = 99, c = 222; int *p = & amp;a; //Define pointer variable *p = b; //Modify the data in memory through pointer variables c = *p; //Get data on memory through pointer variable printf("%d, %d, %d, %d\ ", a, b, c, *p); return 0; }
*p represents the data in a, which is equivalent to a. You can assign another piece of data to it, or you can assign it to another variable.
*
has different functions in different scenarios: *
can be used in the definition of pointer variables to indicate that this is a pointer variable to distinguish it from ordinary variables; use Adding *
in front of a pointer variable means getting the data pointed to by the pointer, or it means the data pointed to by the pointer itself. In other words, the meaning of *
when defining pointer variables is completely different from the meaning of *
when using pointer variables.
Pointer variables can also appear in any expression where ordinary variables can appear, for example:
int x, y, *px = & amp;x, *py = & amp;y; y = *px + 5; //Indicates adding 5 to the content of x and assigning it to y. *px + 5 is equivalent to (*px) + 5 y = + + *px; //Add 1 to the content of px and assign it to y. + + *px is equivalent to + + (*px) y = *px + + ; //Equivalent to y=*(px + + ) py = px; //Assign the value of one pointer to another pointer
Think about it: the value of a pointer variable is the address of a certain piece of data. Such data can be an array, a string, a function, or another ordinary variable or a pointer variable. So what does the following code mean?
int *p; int a=5; p= &a; printf("%d",*p); int **p;//What does this mean?
Finally, to start the next lesson, everyone goes back and tries these three codes.
Before running, think about which one can exchange the values of a and b (must think about it first)
If the results are different from what you expected, think about why they are different.
If it’s the same, congratulations to you. (Better than me, I couldn’t tell the difference before)
//The first one #include <stdio.h> #include <stdlib.h> void Exc(int a,int b); int main() {<!-- --> int a=10,b=5; Exc(a,b); printf("%d,%d",a,b); return 0; } void Exc(int a,int b) {<!-- --> int temp; temp=a; a=b; b=temp; } //the second #include <stdio.h> #include <stdlib.h> void Exc(int *a,int *b); int main() {<!-- --> int a=10,b=5; Exc( & amp;a, & amp;b); printf("%d,%d",a,b); return 0; } void Exc(int *a,int *b) {<!-- --> int temp; temp=*a; *a=*b; *b=temp; } //The third #include <stdio.h> #include <stdlib.h> void Exc(int *a,int *b); int main() {<!-- --> int a=10,b=5; Min( & amp;a, & amp;b); printf("%d,%d",a,b); return 0; } void Exc(int *a,int *b) {<!-- --> int *temp; temp=a; a=b; b=temp; }